A Third Shot of Espressif: Flashing an Espressif ESP01s with an arduino using CLion and PlatformIO
Written on August 18th, 2022 by Sean Webster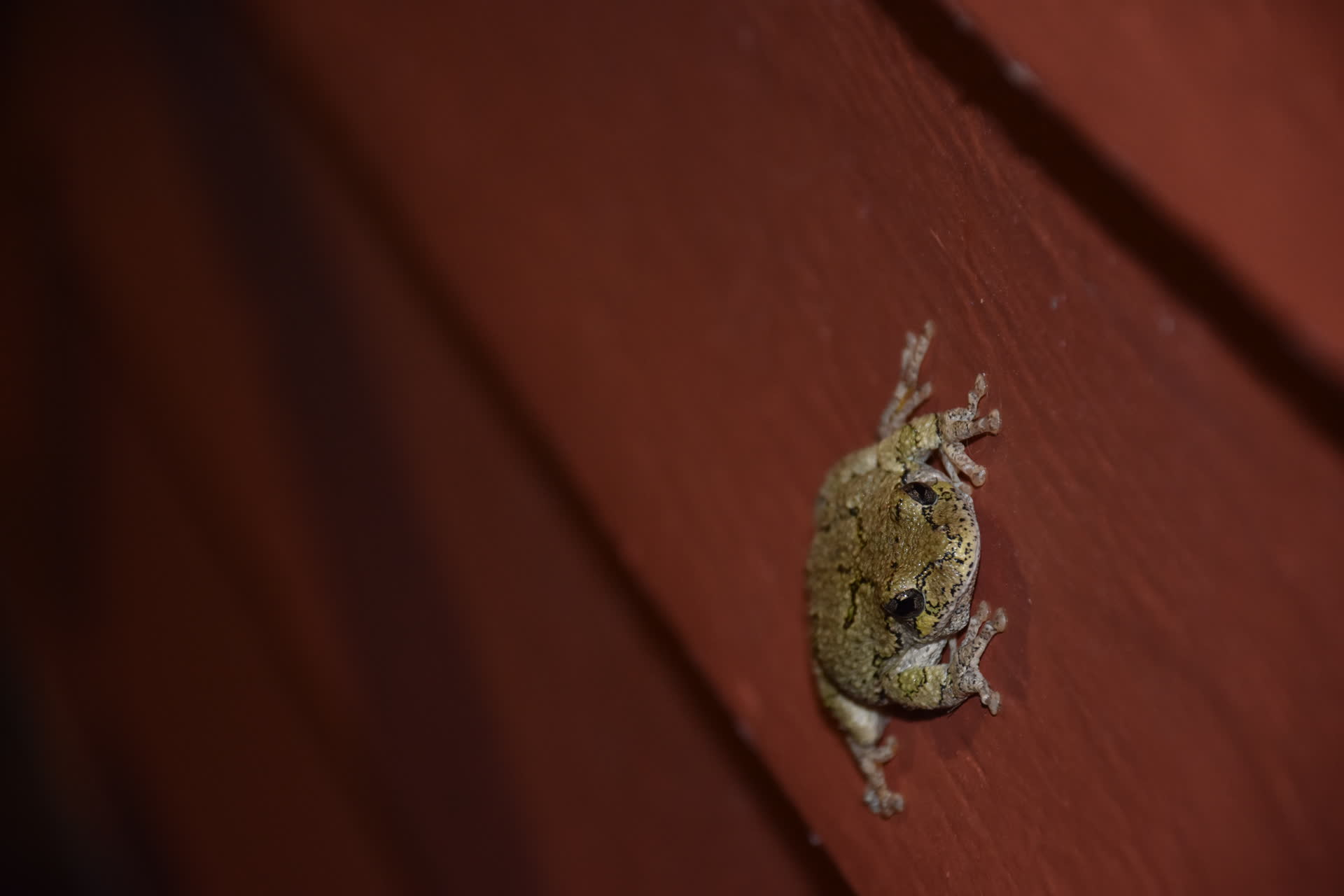
Picture: Gray tree frog
I could have named this something different. I did not.
Setting up PlatformIO with Clion
PlatformIO has documentation here, but I will walk through it.
- Open the CLion plugin menu using
ctrl+shift+A
and typeplugins
- Install the platformIO plugin, by searching for
PlatformIO
Configuring PlatformIO with CLion
PlatformIO automatically downloads configurations for a project if it doesn’t already have them.
- Create a new PlatformIO project:
File->New->Project...
- Select
PlatformIO
->Espressif
->Espressif Generic ESP8266 ESP-01 1M
->esp8266-rtos-sdk
Note: Make sure to check the onboard memory, and that it matches
The PlatformIO project should be configured
Setting up an existing project
PlatformIO is nice enough to supply us with examples at
https://github.com/platformio/platform-espressif8266
. Clone that baby wherever you want, and we can get started.Open your blinky folder example folder in clion
platform-espressif8266\examples\esp8266-rtos-sdk-blink
and create a clion project out of it
- The default blinky project is set up to blink gpio16. We don’t have that, and that’s not where our onboard LED is. So, we’re going to replace
void task_blink(void* ignore)
{
gpio16_output_conf();
while(true) {
gpio16_output_set(0);
vTaskDelay(1000/portTICK_RATE_MS);
gpio16_output_set(1);
vTaskDelay(1000/portTICK_RATE_MS);
}
vTaskDelete(NULL);
}
with
void task_blink(void* ignore)
{
GPIO_ConfigTypeDef config = {.GPIO_Pin = GPIO_OUTPUT_IO_0,
.GPIO_Mode = GPIO_Mode_Output,
.GPIO_Pullup = GPIO_PullUp_DIS,
.GPIO_IntrType = GPIO_PIN_INTR_DISABLE};
gpio_config(&config);
GPIO_OUTPUT_SET(GPIO_OUTPUT_IO_0, 0);
while(true) {
GPIO_OUTPUT_SET(GPIO_OUTPUT_IO_0, 0);
vTaskDelay(1000/portTICK_RATE_MS);
GPIO_OUTPUT_SET(GPIO_OUTPUT_IO_0, 1);
vTaskDelay(1000/portTICK_RATE_MS);
}
vTaskDelete(NULL);
}
so our onboard LED will blink.
- We’re also going to want to remove the build actions that Clion has attached to the PlatformIO actions. We want go to the action dropdown in the menu, and select
Edit Configurations...
- For each of both the
Debug
andUpload
options, remove the build stage by selectingbuild
and hit the-
button.
Building and Flashing
- Select the
PlatformIO Upload
build option, and hit the play button. If all goes as planned, your build should and your board should be flashed
Note: PlatformIO automatically detects upload port by default. You can configure a custom port using upload_port
option in platformio.ini